posted 28 September 2018
In previous posts, I have described my effort to give time, memory and relative heading super-powers to Wall-E2, my autonomous wall-following robot. This posts describes a helper class I created to allow Wall-E2 to periodically write its current operating state to FRAM memory, for later readout by his human master(s), and a small test program to verify proper operation of the helper class.
My current conception of Wall-E2’s operational state consists of the current time/date, its tracking mode and submode, and the current left, right, and forward distances, and the current battery voltage. These parameters have been encapsulated in a CFramStatePacket class with methods for writing state packets to FRAM and reading them back out again. The complete code for this class is shown below. Note that all the class code is contained in just one file – FramPacket.h. There is no associated .cpp file, as I didn’t think that was necessary.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 |
/* CCFRAMStatePacket class. The CFRAMStatePacket class is intended to encapsulate code associated with writing Wall-E2's telemetry to FRAM and reading it back again. This class uses the Adafruit_FRAM_I2C library to actually communicate with the FRAM via I2C. Methods are provided to set up a pointer to the Adafruit_FRAM_I2C object, write/read telemetry packets to/from the FRAM, and print packets to the serial console provided by the calling program. This class assumes the calling program calls Serial.begin() and also provides a StreamEx object called mySerial (typically 'StreamEx mySerial = Serial;'). It also assumes that the reference to this code file is placed *after* the references to Wire.h, Adafruit_I2C.h, and PrintEx.h, and the instantiations of StreamEx MySerial and Adafruit_FRAM_I2C fram. The typical setup would be something like: #include <Wire.h> #include "Adafruit_FRAM_I2C.h" #include <RTClib.h>; #include <time.h>; #include <PrintEx.h> #include <elapsedMillis/elapsedMillis.h> #include <Time-master/time.h> //#include <math.h> StreamEx mySerial = Serial; elapsedMillis mSecSinceLastPacketWrite; Adafruit_FRAM_I2C fram = Adafruit_FRAM_I2C(); const int FIRST_PKT_STORAGE_ADDR = 2;//addr 0/1 reserved for nextFramWriteAddr int nextFramWriteAddr = FIRST_PKT_STORAGE_ADDR; //writing starts with this address int prevDispFramEndAddr = FIRST_PKT_STORAGE_ADDR; //optional readback starts here const int NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION = 0; //the next free address is written to this address //FramPacket.h holds 'inline' definition of CFRAMStatePacket class //this has to come after #include "Adafruit_FRAM_I2C.h" and Adafruit_FRAM_I2C fram = Adafruit_FRAM_I2C(); #include "FramPacket.h" CFRAMStatePacket packet = CFRAMStatePacket(&fram); //this object is used for all FRAM transactions //fwd declaration required to avoid '... does not name a type' error CFRAMStatePacket GetNextSimFRAMPacket(CFRAMStatePacket pkt); [rest of program...] */ #pragma once #ifndef FRAMSTATEPACKET_H #define FRAMSTATEPACKET_H #include "Adafruit_FRAM_I2C.h" //needed for pointer forward declaration const char daysOfTheWeek[7][12] = { "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" }; class CFRAMStatePacket { public: uint32_t unixtime; byte mode; byte submode; byte leftdist; byte rightdist; byte fwddist; byte batv; const static int packet_size = 9; bool bFramReady = false; //added 09/27/18 private: Adafruit_FRAM_I2C* pfram; public: CFRAMStatePacket(uint32_t unixtime = 0, byte mode = 0, byte submode = 0, byte leftdist = 0, byte rightdist = 0, byte fwddist = 0, byte batv = 0); CFRAMStatePacket(Adafruit_FRAM_I2C* pfram, uint32_t unixtime = 0, byte mode = 0, byte submode = 0, byte leftdist = 0, byte rightdist = 0, byte fwddist = 0, byte batv = 0); void Print(); int Read(int addr); //reads packet from FRAM; returns next write addr int Write(int addr); //writes packet to FRAM; returns next read addr void SetFramPointer(Adafruit_FRAM_I2C* ptr); int GetFramPointer(); void PrintHumanReadable(); //displays date & time vs unixtime void CFRAMStatePacket::GetDateTimeStringFromUnixTime(char* str); }; int CFRAMStatePacket::GetFramPointer() { return (int)pfram; } void CFRAMStatePacket::SetFramPointer(Adafruit_FRAM_I2C* ptr) { pfram = ptr; } CFRAMStatePacket::CFRAMStatePacket(uint32_t unixtime = 0, byte mode = 0, byte submode, byte leftdist = 0, byte rightdist = 0, byte fwddist = 0, byte batv = 0) { //mySerial.printf("In CFRAMStatePacket default constructor\n"); } CFRAMStatePacket::CFRAMStatePacket(Adafruit_FRAM_I2C* ptr, uint32_t unixtime = 0, byte mode = 0, byte submode, byte leftdist = 0, byte rightdist = 0, byte fwddist = 0, byte batv = 0) { pfram = ptr; } void CFRAMStatePacket::Print() { mySerial.printf("%lu\t%d\t%d\t%d\t%d\t%d\t%d\n", unixtime, mode, submode, leftdist, rightdist, fwddist, batv); } int CFRAMStatePacket::Write(int addr) { //Provenance: 09/09/18 G. Frank Paynter //Purpose: Write a complete state packet to the FRAM at the specified address // The telemetry packet is formatted as a 9 - byte packet : // Time: 4 - bytes // mode / submode : 1 byte // left distance : 1 byte // right distance : 1 byte // forward distance : 1 byte // battery voltage above 5V : 1 byte //Inputs: // addr = FRAM address to be written to //Outputs: // addr = address to be used for next FRAM write //Plan: // Step1: write each packet component to FRAM at addr, updating addr each time //Notes: //date/time - 4 bytes unsigned long writeval = (unsigned long)unixtime; int numbyteswritten = pfram->FRAM_I2C_writeAnything(addr, writeval); unsigned long readval; pfram->FRAM_I2C_readAnything(addr, readval); addr += numbyteswritten; //mode/submode - 1 bytes byte combomode = (byte)(10 * mode + submode); numbyteswritten = pfram->FRAM_I2C_writeAnything(addr, combomode); addr += numbyteswritten; //left dist - 1 bytes numbyteswritten = pfram->FRAM_I2C_writeAnything(addr, leftdist); addr += numbyteswritten; //right dist - 1 bytes numbyteswritten = pfram->FRAM_I2C_writeAnything(addr, rightdist); addr += numbyteswritten; //forward dist - 1 bytes numbyteswritten = pfram->FRAM_I2C_writeAnything(addr, fwddist); addr += numbyteswritten; //batV - 1 bytes numbyteswritten = pfram->FRAM_I2C_writeAnything(addr, batv); addr += numbyteswritten; return addr; } int CFRAMStatePacket::Read(int addr) { //Provenance: 09/09/18 G. Frank Paynter //Purpose: read a complete state packet from the FRAM at the specified address // The telemetry packet is formatted as a 9 - byte packet : // Time: 4 - bytes // mode / submode : 1 byte // left distance : 1 byte // right distance : 1 byte // forward distance : 1 byte // battery voltage above 5V : 1 byte //Inputs: // addr = FRAM address to be read from //Outputs: // addr = address to be used for next FRAM read //Plan: // Step1: read each packet component from FRAM at addr, updating addr each time //Notes: //mySerial.printf("In pkt.Read with address %d and Adafruit_FRAM_I2C* = %d\n", addr,pfram); //unixtime uint32_t utime; pfram->FRAM_I2C_readAnything(addr, unixtime); addr += sizeof( utime); //mode, submode byte combomode; pfram->FRAM_I2C_readAnything(addr, combomode); mode = combomode / 10; //integer truncation OK submode = combomode - 10 * mode; addr++; //distances pfram->FRAM_I2C_readAnything(addr, leftdist); addr++; pfram->FRAM_I2C_readAnything(addr, rightdist); addr++; pfram->FRAM_I2C_readAnything(addr, fwddist); addr++; //batt voltage pfram->FRAM_I2C_readAnything(addr, batv); addr++; return addr; } void CFRAMStatePacket::PrintHumanReadable() //displays date & time vs unixtime { //Provenance: 10/01/18 G. Frank Paynter //Purpose: print a complete state packet with the uint32_t unixtime property // replaced by a human-readable date-time format. //Inputs: None //Outputs: // packet contents sent to serial port, with date/time instead of unixtime //Plan: // Step1: print out the human-readable date/time instead of unixtime // Step2: print the rest out as normal //Notes: //Step1: print out the human-readable date/time instead of unixtime char datestr[40]; memset(datestr, 0, sizeof(datestr)); GetDateTimeStringFromUnixTime(datestr); Serial.print(datestr); //can't use mySerial.printf() for some reason //Step2: print the rest out as normal mySerial.printf("\t%d\t%d\t%d\t%d\t%d\t%d\n", mode, submode, leftdist, rightdist, fwddist, batv); } void CFRAMStatePacket::GetDateTimeStringFromUnixTime(char* datestr) { time_t utime = (time_t)unixtime; int mydayofweek = weekday(utime) - 1; //returns 1 for Sunday, 7 for Saturday mydayofweek = (mydayofweek < 0) ? 0 : mydayofweek; //guard for return of 0 from weekday() int myday = day(utime); int mymonth = month(utime); int myyear = year(utime); int myhour = hour(utime); int mymin = minute(utime); int mysec = second(utime); char* dayofweek = daysOfTheWeek[mydayofweek]; sprintf(datestr, "%02d:%02d:%02d %02d/%02d/%4d", myhour, mymin, mysec, mymonth, myday, myyear ); } #endif |
To test my new CFRAMStatePacket class, I created a small test program that periodically writes simulated state packets to FRAM using the helper class methods, and optionally (if the user creates an interrupt by grounding the appropriate pin) reads them back out again. This program is designed to run on an Arduino Mega 2560. If a Uno is used, the interrupt pin number would have to be changed.
The test code also looks for a low on the CLEAR_FRAM_PIN (Pin 3) on startup. If it finds one, it will clear NUM_FRAM_BYTES_TO_CLEAR (currently 2000) FRAM bytes and then read them back out again, byte-by-byte. Otherwise, the program will continue storing state packets where it left off the last time it was powered up. Here’s the test code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 |
/* Name: TelemPacketsToFromFRAM.ino Created: 9/9/2018 9:47:30 PM Author: DESKTOP-0KFED77\Frank The purpose of this project is to develop the code needed to write encoded telemetry packets to the FRAM, and optionally read them back out again in decoded form. The telemetry packet is formatted as a 10-byte packet: Time: 4-bytes mode/submode: 1 byte left distance: 1 byte right distance: 1 byte forward distance: 1 byte battery voltage above 5V: 1 byte This project makes use of the CFRAMStatePacket class, which is completely defined in FramPacket.h (no .cpp file - just the .h). This class contains methods to read and write telemetry packets from/to an Adafruit FRAM breakout board, accessible through the Adafruit_FRAM_I2C library To use the CFRAMStatePacket class, an object is instantiated using a constructor that takes a single parameter - the address of the Adafruit_FRAM_I2C FRAM driver object. This pointer is then used for all interactions with the FRAM. */ #include <Wire.h> #include "Adafruit_FRAM_I2C.h" #include <RTClib.h>; #include <time.h>; #include <PrintEx.h> #include <elapsedMillis/elapsedMillis.h> #include <Time-master/time.h> //#include <math.h> StreamEx mySerial = Serial; elapsedMillis mSecSinceLastPacketWrite; Adafruit_FRAM_I2C fram = Adafruit_FRAM_I2C(); const int FIRST_PKT_STORAGE_ADDR = 2;//addr 0/1 reserved for nextFramWriteAddr //const int FIRST_PKT_STORAGE_ADDR = 32000;//09/29/18 for rollover debug int nextFramWriteAddr = FIRST_PKT_STORAGE_ADDR; //writing starts with this address int prevDispFramEndAddr = FIRST_PKT_STORAGE_ADDR; //optional readback starts here const int NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION = 0; //the next free address is written to this address const int LAST_AVAIL_PACKET_WRITE_ADDR = 32759; //last packet write address avail in 256kb FRAM //FramPacket.h holds 'inline' definition of CFRAMStatePacket class //this has to come after #include "Adafruit_FRAM_I2C.h" and Adafruit_FRAM_I2C fram = Adafruit_FRAM_I2C(); #include "FramPacket.h" CFRAMStatePacket packet = CFRAMStatePacket(&fram); //this object is used for all FRAM transactions //fwd declaration required to avoid '... does not name a type' error CFRAMStatePacket GetNextSimFRAMPacket(CFRAMStatePacket pkt); RTC_DS3231 rtc; char daysOfTheWeek[7][12] = { "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" }; const int InterruptPin = 18; //pin used to trigger a readback of previously written packet const int CLEAR_FRAM_PIN = 3;//holding this low at startup will clear NUM_FRAM_BYTES_TO_CLEAR FRAM bytes const int NUM_FRAM_BYTES_TO_CLEAR = 2000; //don't clear the whole thing - takes too long const int NUM_PACKETS_TO_DISPLAY = 10; //for 1st time packet display //const unsigned long PACKET_WRITE_INTERVAL_MSEC = 1000; //once/sec for debug const unsigned long PACKET_WRITE_INTERVAL_MSEC = 250; //4/sec for debug bool InSetup = true; //used to prevent interrupts during setup bool bFirstPacketWrite = true;//used to print packet header the first time through bool bInterrupt = false;//set true in myISR, reset in PACKET_WRITE_INTERVAL_MSEC section of loop() //These are the operation modes used by the Wall-E2 robot. They are used here to //simulate normal mode variations to verify proper encode/decode enum OpModes { MODE_NONE = 0, //04/04/17 chg from MODE_DEFAULT and moved to top (zero) position MODE_CHARGING, MODE_IRHOMING, MODE_WALLFOLLOW, MODE_DEADBATTERY //added 01/16/18 to handle dead battery case }; //01/05/16 added for open-corner following supp const int DIST_AVG_WINDOW_SIZE = 3; enum WallTrackingCases { TRACKING_LEFT = 1, TRACKING_RIGHT, TRACKING_NEITHER }; enum OpModes simOpMode = MODE_NONE; enum WallTrackingCases simTrack = TRACKING_LEFT; byte simLeftDist = 0; byte simRightDist = 0; byte simForwardDist = 0; uint32_t simTime = 0; void setup() { Serial.begin(115200); //initialize Adafruit DS3231 Real Time Clock mySerial.printf("Initializing RTC...\n"); if (!rtc.begin()) { Serial.println("Couldn't find RTC"); while (1); } if (rtc.lostPower()) { DateTime dt = DateTime(F(__DATE__), F(__TIME__)); //__DATE__ & __TIME__ are environment variables) int mydayofweek = dt.dayOfTheWeek(); //returns 0 for Sunday, 7 for Saturday int mymonth = dt.month(); int myday = dt.day(); int myyear = dt.year(); int myhour = dt.hour(); int mymin = dt.minute(); int mysec = dt.second(); long unixtime = dt.unixtime(); char* dayofweek = daysOfTheWeek[mydayofweek]; mySerial.printf("RTC lost power - setting to datetime of last compile %ld (%s %4d/%02d/%02d at %02d:%02d:%02d)\n", dt.unixtime(), daysOfTheWeek[dt.dayOfTheWeek()], dt.year(), dt.month(), dt.day(), dt.hour(), dt.minute(), dt.second()); rtc.adjust(dt); } //initialize FRAM - this reads/checks FRAM device IDs to confirm valid connection mySerial.printf("Initializing FRAM...\n"); if (fram.begin()) // you can stick the new i2c addr in here, e.g. begin(0x51); { Serial.println("Found I2C FRAM"); } else { Serial.println("I2C FRAM not identified ... check your connections?\r\n"); Serial.println("Will continue in case this processor doesn't support repeated start\r\n"); } // Read the stored nextFramWriteAddr value from the first two bytes fram.FRAM_I2C_readAnything(NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION, nextFramWriteAddr); mySerial.printf("read nextFramWriteAddr %d from address %d\n", nextFramWriteAddr, NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION); pinMode(InterruptPin, INPUT_PULLUP); pinMode(CLEAR_FRAM_PIN, INPUT_PULLUP); int intnum = digitalPinToInterrupt(InterruptPin); mySerial.printf("Using interrupt number %d, pin %d\n", intnum, InterruptPin); attachInterrupt(intnum, myISR, FALLING); interrupts(); //to clear the first NUM_FRAM_BYTES_TO_CLEAR FRAM locations, hold this pin low at startup if (digitalRead(CLEAR_FRAM_PIN) == LOW) { Serial.print("Clearing "); Serial.print(NUM_FRAM_BYTES_TO_CLEAR); Serial.println(" FRAM addresses..."); byte clearval = 0; for (size_t i = 0; i < NUM_FRAM_BYTES_TO_CLEAR; i++) { fram.FRAM_I2C_writeAnything(i, clearval); } mySerial.printf("Setting NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION (%d) to FIRST_PKT_STORAGE_ADDR (%d)\n", NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION, FIRST_PKT_STORAGE_ADDR); fram.FRAM_I2C_writeAnything(NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION, FIRST_PKT_STORAGE_ADDR); nextFramWriteAddr = FIRST_PKT_STORAGE_ADDR; //this is the only place it gets set - otherwise it is retrieved from the FRAM at address 1 //now read them back DisplayFRAMBytes(NUM_FRAM_BYTES_TO_CLEAR); } else { int displayendaddr = NUM_PACKETS_TO_DISPLAY * CFRAMStatePacket::packet_size + FIRST_PKT_STORAGE_ADDR; DisplayFRAMPackets(FIRST_PKT_STORAGE_ADDR, displayendaddr); } Serial.print("Enabling ISR, Entering Loop()"); mSecSinceLastPacketWrite = 0; //reset just before loop() InSetup = false; //enable power-down ISR bInterrupt = false; } void loop() { if (mSecSinceLastPacketWrite >= PACKET_WRITE_INTERVAL_MSEC) { mSecSinceLastPacketWrite -= PACKET_WRITE_INTERVAL_MSEC; //check for interrupt. If active, display packets written since last time if (bInterrupt) { //09/29/18 have to watch for prevDispFramEndAddr > nextFramWriteAddr if (prevDispFramEndAddr > nextFramWriteAddr) { prevDispFramEndAddr = nextFramWriteAddr; } DisplayFRAMPackets(prevDispFramEndAddr, nextFramWriteAddr); prevDispFramEndAddr = nextFramWriteAddr; //added 09/28/18 bInterrupt = false; } packet = GetNextSimFRAMPacket(packet); //update packet contents if (bFirstPacketWrite) { mySerial.printf("addr\tunixtime\t\tmode\tsubmode\tLdist\tRdist\tFdist\tbatV\n"); bFirstPacketWrite = false; } //check for FRAM address rollover - reset to first write address if necessary if (nextFramWriteAddr >= LAST_AVAIL_PACKET_WRITE_ADDR) { nextFramWriteAddr = FIRST_PKT_STORAGE_ADDR; } mySerial.printf("%d\t", nextFramWriteAddr); //puts fram addr in front of each packet readout packet.Print(); nextFramWriteAddr = packet.Write(nextFramWriteAddr); //actually write the packet to FRAM fram.FRAM_I2C_writeAnything(NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION, nextFramWriteAddr); //update stored nextFramWriteAddr } } //not used, but left here for possible future use void DisplayFRAMTimes(int startaddr, int endaddr) { time_t utime; int numtimes = endaddr / sizeof(utime); mySerial.printf("\nDisplaying first %d stored times starting at %d\n", numtimes, startaddr); for (uint16_t i = 0; i < numtimes; i++) { int addr = startaddr + i * sizeof(utime); //int numbytes = fram.FRAM_I2C_readAnything(addr, utime); int mydayofweek = weekday(utime) - 1; //returns 1 for Sunday, 7 for Saturday mydayofweek = (mydayofweek < 0) ? 0 : mydayofweek; //guard for return of 0 from weekday() int myday = day(utime); int mymonth = month(utime); int myyear = year(utime); int myhour = hour(utime); int mymin = minute(utime); int mysec = second(utime); char* dayofweek = daysOfTheWeek[mydayofweek]; mySerial.printf("read %ld (%s %4d/%02d/%02d at %02d:%02d:%02d) from FRAM location %d\n", utime, dayofweek, myyear, mymonth, myday, myhour, mymin, mysec, addr); } } void DisplayFRAMBytes(int lastAddr) { Serial.print("\nDisplaying memory locations 0 to "); Serial.println(lastAddr); uint8_t value; for (uint16_t a = 0; a < lastAddr; a++) { if ((a % 16) == 0) { Serial.print("\n 0x"); Serial.print(a, HEX); Serial.print(": "); } Serial.print("0x"); if (value < 0x1) Serial.print('0'); Serial.print(value, HEX); Serial.print(" "); } Serial.println(); } void myISR() { bInterrupt = true; } CFRAMStatePacket GetNextSimFRAMPacket(CFRAMStatePacket pkt) { DateTime dt = rtc.now(); pkt.leftdist = GetNextSimLeftDist(pkt.leftdist); pkt.rightdist = GetNextSimRightDist(pkt.rightdist); pkt.fwddist = GetNextSimFwdDist(pkt.leftdist); //uses leftdist to calc fwddist pkt.mode = GetNextSimMode(pkt.mode); pkt.submode = GetNextSimTrack(pkt.submode); pkt.unixtime = (uint32_t)dt.unixtime(); return pkt; } int GetNextSimLeftDist(int leftdist) { //zero to 200 sawtooth int simdist = (leftdist >= 200) ? 0 : ++leftdist; return simdist; } int GetNextSimRightDist(int rightdist) { //200 to zero sawtooth int simdist = (rightdist <= 0) ? 200 : --rightdist; return simdist; } int GetNextSimFwdDist(int leftdist) { //uses leftdist to calc new point on sine curve //zero to 200 sinewave float rads = PI*(float)leftdist/100.f; int simdist = 100 + (int)(100 * sin(rads)); return simdist; } int GetNextSimMode(int opmode) { switch (opmode) { case MODE_NONE: opmode = MODE_CHARGING; break; case MODE_CHARGING: opmode = MODE_IRHOMING; break; case MODE_IRHOMING: opmode = MODE_WALLFOLLOW; break; case MODE_WALLFOLLOW: opmode = MODE_DEADBATTERY; break; case MODE_DEADBATTERY: opmode = MODE_CHARGING; break; default: mySerial.printf("hit default case in GetNextSimMode()\n"); opmode = MODE_NONE; break; } return (int)opmode; } int GetNextSimTrack(int trackmode) { switch (trackmode) { case 0: trackmode = TRACKING_LEFT; break; case TRACKING_LEFT: trackmode = TRACKING_RIGHT; break; case TRACKING_RIGHT: trackmode = TRACKING_NEITHER; break; case TRACKING_NEITHER: trackmode = TRACKING_LEFT; break; default: mySerial.printf("hit default case in GetNextSimTrack()\n"); trackmode = 0; break; } return (int)trackmode; } void DisplayFRAMPackets(int nextreadaddr, int nextwriteaddr) { //Purpose: Read complete state packets from the FRAM between the specified addresses // The telemetry packet is formatted as a 10 - byte packet : // Time: 4 - bytes // mode / submode : 1 byte // left distance : 1 byte // right distance : 1 byte // forward distance : 1 byte // battery voltage above 5V : 1 byte //Inputs: // nextreadaddr = 1st address to be read // nextwriteaddr = next available address for writes //Notes: // 09/28/18 c/o prevDispFramEndAddr = nextwriteaddr - 1; now done in calling pgm int numpackets = 1 + (nextwriteaddr - nextreadaddr - 1) / CFRAMStatePacket::packet_size; mySerial.printf("\ndisplaying %d packets, from address %d to address %d\n", numpackets, nextreadaddr, nextwriteaddr - 1); mySerial.printf("addr\tunixtime\t\tmode\tsubmode\tLdist\tRdist\tFdist\tbatV\n"); for (int i = 0; i < numpackets; i++) { mySerial.printf("%d\t", nextreadaddr); //puts fram addr in front of each packet readout nextreadaddr = packet.Read(nextreadaddr); packet.Print(); } mySerial.printf("---------------- Done!-----------------\n\n"); mySerial.printf("addr\tunixtime\t\tmode\tsubmode\tLdist\tRdist\tFdist\tbatV\n"); } |
And here’s some output from a typical run:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 601 602 603 604 605 606 607 608 609 610 611 612 613 614 615 616 617 618 619 620 621 622 623 624 625 626 627 628 629 630 631 632 633 634 635 636 637 638 639 640 641 642 643 644 645 646 647 648 649 650 651 652 653 654 655 656 657 658 659 660 661 662 663 664 665 666 667 668 669 670 671 672 673 674 675 676 677 678 679 680 681 682 683 684 685 686 687 688 689 690 691 692 693 694 695 696 697 698 699 700 701 702 703 704 705 706 707 708 709 710 711 712 713 714 715 716 717 718 719 720 721 722 723 724 725 726 727 728 729 730 731 732 733 734 735 736 737 738 739 740 741 742 743 744 745 746 747 748 749 750 751 752 753 754 755 756 757 758 759 760 761 762 763 764 765 766 767 768 769 770 771 772 773 774 775 776 777 778 779 780 781 782 783 784 785 786 787 788 789 790 791 792 793 794 795 796 797 798 799 800 801 802 803 804 805 806 807 808 809 810 811 812 813 814 815 816 817 818 819 820 821 822 823 824 825 826 827 828 829 830 831 832 833 834 835 836 837 838 839 840 841 842 843 844 845 846 847 848 849 850 851 852 853 854 855 856 857 858 859 860 861 862 863 864 865 866 867 868 869 870 871 872 873 874 875 876 877 878 879 880 881 882 883 884 885 886 887 888 889 890 891 892 893 894 895 896 897 898 899 900 901 902 903 904 905 906 907 908 909 910 911 912 913 914 915 916 917 918 919 920 921 922 923 924 925 926 927 928 929 930 931 932 933 934 935 936 937 938 939 940 941 942 943 944 945 946 947 948 949 950 951 952 953 954 955 956 957 958 959 960 961 962 963 964 965 966 967 968 969 970 971 972 973 974 975 976 977 978 979 980 981 982 983 984 985 986 987 988 989 990 991 992 993 994 995 996 997 998 999 1000 1001 1002 1003 1004 1005 1006 1007 1008 1009 1010 1011 1012 1013 1014 1015 1016 1017 1018 1019 1020 1021 1022 1023 1024 1025 1026 1027 1028 1029 1030 1031 1032 1033 1034 1035 1036 1037 1038 1039 1040 1041 1042 1043 1044 1045 1046 1047 1048 1049 1050 1051 1052 1053 1054 1055 1056 1057 1058 1059 1060 1061 1062 1063 1064 1065 1066 1067 1068 1069 1070 1071 1072 1073 1074 1075 1076 1077 1078 1079 1080 1081 1082 1083 1084 1085 1086 1087 1088 1089 1090 1091 1092 1093 1094 1095 1096 1097 1098 1099 1100 1101 1102 1103 1104 1105 1106 1107 1108 1109 1110 1111 1112 1113 1114 1115 1116 |
Opening port Port open Initializing RTC... Initializing FRAM... Found I2C FRAM read nextFramWriteAddr 8255 from address 0 Using interrupt number 5, pin 18 displaying 10 packets, from address 2 to address 91 addr unixtime mode submode Ldist Rdist Fdist batV 2 1538191317 2 1 166 35 13 0 11 1538191317 3 2 167 34 14 0 20 1538191318 4 3 168 33 16 0 29 1538191318 1 1 169 32 18 0 38 1538191318 2 2 170 31 20 0 47 1538191318 3 3 171 30 21 0 56 1538191319 4 1 172 29 23 0 65 1538191319 1 2 173 28 25 0 74 1538191319 2 3 174 27 28 0 83 1538191319 3 1 175 26 30 0 ---------------- Done!----------------- addr unixtime mode submode Ldist Rdist Fdist batV Enabling ISR, Entering Loop()addr unixtime mode submode Ldist Rdist Fdist batV 8255 1538191560 4 2 176 25 32 0 8264 1538191560 1 3 177 24 34 0 8273 1538191560 2 1 178 23 37 0 8282 1538191561 3 2 179 22 39 0 8291 1538191561 4 3 180 21 42 0 8300 1538191561 1 1 181 20 44 0 8309 1538191561 2 2 182 19 47 0 8318 1538191562 3 3 183 18 50 0 8327 1538191562 4 1 184 17 52 0 8336 1538191562 1 2 185 16 55 0 8345 1538191562 2 3 186 15 58 0 8354 1538191563 3 1 187 14 61 0 8363 1538191563 4 2 188 13 64 0 8372 1538191563 1 3 189 12 67 0 8381 1538191563 2 1 190 11 70 0 8390 1538191564 3 2 191 10 73 0 8399 1538191564 4 3 192 9 76 0 8408 1538191564 1 1 193 8 79 0 8417 1538191564 2 2 194 7 82 0 8426 1538191565 3 3 195 6 85 0 8435 1538191565 4 1 196 5 88 0 8444 1538191565 1 2 197 4 91 0 8453 1538191565 2 3 198 3 94 0 8462 1538191566 3 1 199 2 97 0 8471 1538191566 4 2 200 1 100 0 8480 1538191566 1 3 0 0 100 0 8489 1538191566 2 1 1 200 103 0 8498 1538191567 3 2 2 199 106 0 8507 1538191567 4 3 3 198 109 0 8516 1538191567 1 1 4 197 112 0 8525 1538191567 2 2 5 196 115 0 8534 1538191568 3 3 6 195 118 0 8543 1538191568 4 1 7 194 121 0 8552 1538191568 1 2 8 193 124 0 8561 1538191568 2 3 9 192 127 0 8570 1538191569 3 1 10 191 130 0 8579 1538191569 4 2 11 190 133 0 8588 1538191569 1 3 12 189 136 0 8597 1538191569 2 1 13 188 139 0 8606 1538191570 3 2 14 187 142 0 8615 1538191570 4 3 15 186 145 0 8624 1538191570 1 1 16 185 148 0 8633 1538191570 2 2 17 184 150 0 8642 1538191571 3 3 18 183 153 0 8651 1538191571 4 1 19 182 156 0 8660 1538191571 1 2 20 181 158 0 8669 1538191571 2 3 21 180 161 0 8678 1538191572 3 1 22 179 163 0 8687 1538191572 4 2 23 178 166 0 8696 1538191572 1 3 24 177 168 0 8705 1538191572 2 1 25 176 170 0 8714 1538191573 3 2 26 175 172 0 8723 1538191573 4 3 27 174 175 0 8732 1538191573 1 1 28 173 177 0 8741 1538191573 2 2 29 172 179 0 8750 1538191574 3 3 30 171 180 0 8759 1538191574 4 1 31 170 182 0 8768 1538191574 1 2 32 169 184 0 8777 1538191574 2 3 33 168 186 0 8786 1538191575 3 1 34 167 187 0 8795 1538191575 4 2 35 166 189 0 8804 1538191575 1 3 36 165 190 0 8813 1538191575 2 1 37 164 191 0 8822 1538191576 3 2 38 163 192 0 8831 1538191576 4 3 39 162 194 0 8840 1538191576 1 1 40 161 195 0 8849 1538191576 2 2 41 160 196 0 8858 1538191577 3 3 42 159 196 0 8867 1538191577 4 1 43 158 197 0 8876 1538191577 1 2 44 157 198 0 8885 1538191577 2 3 45 156 198 0 8894 1538191578 3 1 46 155 199 0 8903 1538191578 4 2 47 154 199 0 8912 1538191578 1 3 48 153 199 0 8921 1538191578 2 1 49 152 199 0 8930 1538191579 3 2 50 151 200 0 8939 1538191579 4 3 51 150 199 0 8948 1538191579 1 1 52 149 199 0 8957 1538191579 2 2 53 148 199 0 8966 1538191580 3 3 54 147 199 0 8975 1538191580 4 1 55 146 198 0 8984 1538191580 1 2 56 145 198 0 8993 1538191580 2 3 57 144 197 0 9002 1538191581 3 1 58 143 196 0 9011 1538191581 4 2 59 142 196 0 9020 1538191581 1 3 60 141 195 0 9029 1538191581 2 1 61 140 194 0 9038 1538191582 3 2 62 139 192 0 9047 1538191582 4 3 63 138 191 0 9056 1538191582 1 1 64 137 190 0 9065 1538191582 2 2 65 136 189 0 9074 1538191583 3 3 66 135 187 0 9083 1538191583 4 1 67 134 186 0 9092 1538191583 1 2 68 133 184 0 9101 1538191583 2 3 69 132 182 0 9110 1538191584 3 1 70 131 180 0 9119 1538191584 4 2 71 130 179 0 9128 1538191584 1 3 72 129 177 0 9137 1538191584 2 1 73 128 175 0 9146 1538191585 3 2 74 127 172 0 9155 1538191585 4 3 75 126 170 0 9164 1538191585 1 1 76 125 168 0 9173 1538191585 2 2 77 124 166 0 9182 1538191586 3 3 78 123 163 0 9191 1538191586 4 1 79 122 161 0 9200 1538191586 1 2 80 121 158 0 9209 1538191586 2 3 81 120 156 0 9218 1538191587 3 1 82 119 153 0 9227 1538191587 4 2 83 118 150 0 9236 1538191587 1 3 84 117 148 0 9245 1538191587 2 1 85 116 145 0 9254 1538191588 3 2 86 115 142 0 9263 1538191588 4 3 87 114 139 0 9272 1538191588 1 1 88 113 136 0 9281 1538191588 2 2 89 112 133 0 9290 1538191589 3 3 90 111 130 0 9299 1538191589 4 1 91 110 127 0 9308 1538191589 1 2 92 109 124 0 9317 1538191589 2 3 93 108 121 0 9326 1538191590 3 1 94 107 118 0 9335 1538191590 4 2 95 106 115 0 9344 1538191590 1 3 96 105 112 0 9353 1538191590 2 1 97 104 109 0 9362 1538191591 3 2 98 103 106 0 9371 1538191591 4 3 99 102 103 0 9380 1538191591 1 1 100 101 100 0 9389 1538191591 2 2 101 100 97 0 9398 1538191592 3 3 102 99 94 0 9407 1538191592 4 1 103 98 91 0 9416 1538191592 1 2 104 97 88 0 9425 1538191592 2 3 105 96 85 0 9434 1538191593 3 1 106 95 82 0 9443 1538191593 4 2 107 94 79 0 9452 1538191593 1 3 108 93 76 0 9461 1538191593 2 1 109 92 73 0 9470 1538191594 3 2 110 91 70 0 9479 1538191594 4 3 111 90 67 0 9488 1538191594 1 1 112 89 64 0 9497 1538191594 2 2 113 88 61 0 9506 1538191595 3 3 114 87 58 0 9515 1538191595 4 1 115 86 55 0 9524 1538191595 1 2 116 85 52 0 9533 1538191595 2 3 117 84 50 0 9542 1538191596 3 1 118 83 47 0 9551 1538191596 4 2 119 82 44 0 9560 1538191596 1 3 120 81 42 0 9569 1538191596 2 1 121 80 39 0 9578 1538191597 3 2 122 79 37 0 9587 1538191597 4 3 123 78 34 0 9596 1538191597 1 1 124 77 32 0 9605 1538191597 2 2 125 76 30 0 9614 1538191598 3 3 126 75 28 0 9623 1538191598 4 1 127 74 25 0 9632 1538191598 1 2 128 73 23 0 9641 1538191598 2 3 129 72 21 0 9650 1538191599 3 1 130 71 20 0 9659 1538191599 4 2 131 70 18 0 9668 1538191599 1 3 132 69 16 0 9677 1538191599 2 1 133 68 14 0 9686 1538191600 3 2 134 67 13 0 9695 1538191600 4 3 135 66 11 0 9704 1538191600 1 1 136 65 10 0 9713 1538191600 2 2 137 64 9 0 9722 1538191601 3 3 138 63 8 0 9731 1538191601 4 1 139 62 6 0 9740 1538191601 1 2 140 61 5 0 9749 1538191601 2 3 141 60 4 0 9758 1538191602 3 1 142 59 4 0 9767 1538191602 4 2 143 58 3 0 9776 1538191602 1 3 144 57 2 0 9785 1538191602 2 1 145 56 2 0 9794 1538191603 3 2 146 55 1 0 9803 1538191603 4 3 147 54 1 0 9812 1538191603 1 1 148 53 1 0 9821 1538191603 2 2 149 52 1 0 9830 1538191604 3 3 150 51 0 0 9839 1538191604 4 1 151 50 1 0 9848 1538191604 1 2 152 49 1 0 9857 1538191604 2 3 153 48 1 0 9866 1538191605 3 1 154 47 1 0 9875 1538191605 4 2 155 46 2 0 9884 1538191605 1 3 156 45 2 0 9893 1538191605 2 1 157 44 3 0 9902 1538191606 3 2 158 43 4 0 9911 1538191606 4 3 159 42 4 0 9920 1538191606 1 1 160 41 5 0 9929 1538191606 2 2 161 40 6 0 9938 1538191607 3 3 162 39 8 0 9947 1538191607 4 1 163 38 9 0 9956 1538191607 1 2 164 37 10 0 9965 1538191607 2 3 165 36 11 0 9974 1538191608 3 1 166 35 13 0 9983 1538191608 4 2 167 34 14 0 9992 1538191608 1 3 168 33 16 0 10001 1538191608 2 1 169 32 18 0 10010 1538191609 3 2 170 31 20 0 10019 1538191609 4 3 171 30 21 0 10028 1538191609 1 1 172 29 23 0 10037 1538191609 2 2 173 28 25 0 10046 1538191610 3 3 174 27 28 0 10055 1538191610 4 1 175 26 30 0 10064 1538191610 1 2 176 25 32 0 10073 1538191610 2 3 177 24 34 0 10082 1538191611 3 1 178 23 37 0 10091 1538191611 4 2 179 22 39 0 10100 1538191611 1 3 180 21 42 0 10109 1538191611 2 1 181 20 44 0 10118 1538191612 3 2 182 19 47 0 10127 1538191612 4 3 183 18 50 0 10136 1538191612 1 1 184 17 52 0 10145 1538191612 2 2 185 16 55 0 10154 1538191613 3 3 186 15 58 0 10163 1538191613 4 1 187 14 61 0 10172 1538191613 1 2 188 13 64 0 10181 1538191613 2 3 189 12 67 0 10190 1538191614 3 1 190 11 70 0 10199 1538191614 4 2 191 10 73 0 10208 1538191614 1 3 192 9 76 0 10217 1538191614 2 1 193 8 79 0 10226 1538191615 3 2 194 7 82 0 10235 1538191615 4 3 195 6 85 0 10244 1538191615 1 1 196 5 88 0 10253 1538191615 2 2 197 4 91 0 10262 1538191615 3 3 198 3 94 0 10271 1538191616 4 1 199 2 97 0 10280 1538191616 1 2 200 1 100 0 10289 1538191616 2 3 0 0 100 0 10298 1538191616 3 1 1 200 103 0 10307 1538191617 4 2 2 199 106 0 10316 1538191617 1 3 3 198 109 0 10325 1538191617 2 1 4 197 112 0 10334 1538191617 3 2 5 196 115 0 10343 1538191618 4 3 6 195 118 0 10352 1538191618 1 1 7 194 121 0 10361 1538191618 2 2 8 193 124 0 10370 1538191618 3 3 9 192 127 0 10379 1538191619 4 1 10 191 130 0 10388 1538191619 1 2 11 190 133 0 10397 1538191619 2 3 12 189 136 0 10406 1538191619 3 1 13 188 139 0 10415 1538191620 4 2 14 187 142 0 10424 1538191620 1 3 15 186 145 0 10433 1538191620 2 1 16 185 148 0 10442 1538191620 3 2 17 184 150 0 10451 1538191621 4 3 18 183 153 0 10460 1538191621 1 1 19 182 156 0 10469 1538191621 2 2 20 181 158 0 10478 1538191621 3 3 21 180 161 0 10487 1538191622 4 1 22 179 163 0 10496 1538191622 1 2 23 178 166 0 10505 1538191622 2 3 24 177 168 0 10514 1538191622 3 1 25 176 170 0 10523 1538191623 4 2 26 175 172 0 10532 1538191623 1 3 27 174 175 0 10541 1538191623 2 1 28 173 177 0 10550 1538191623 3 2 29 172 179 0 10559 1538191624 4 3 30 171 180 0 10568 1538191624 1 1 31 170 182 0 10577 1538191624 2 2 32 169 184 0 10586 1538191624 3 3 33 168 186 0 10595 1538191625 4 1 34 167 187 0 10604 1538191625 1 2 35 166 189 0 10613 1538191625 2 3 36 165 190 0 10622 1538191625 3 1 37 164 191 0 10631 1538191626 4 2 38 163 192 0 10640 1538191626 1 3 39 162 194 0 10649 1538191626 2 1 40 161 195 0 10658 1538191626 3 2 41 160 196 0 10667 1538191627 4 3 42 159 196 0 10676 1538191627 1 1 43 158 197 0 10685 1538191627 2 2 44 157 198 0 10694 1538191627 3 3 45 156 198 0 10703 1538191628 4 1 46 155 199 0 10712 1538191628 1 2 47 154 199 0 10721 1538191628 2 3 48 153 199 0 10730 1538191628 3 1 49 152 199 0 10739 1538191629 4 2 50 151 200 0 10748 1538191629 1 3 51 150 199 0 10757 1538191629 2 1 52 149 199 0 10766 1538191629 3 2 53 148 199 0 10775 1538191630 4 3 54 147 199 0 10784 1538191630 1 1 55 146 198 0 10793 1538191630 2 2 56 145 198 0 10802 1538191630 3 3 57 144 197 0 10811 1538191631 4 1 58 143 196 0 10820 1538191631 1 2 59 142 196 0 10829 1538191631 2 3 60 141 195 0 10838 1538191631 3 1 61 140 194 0 10847 1538191632 4 2 62 139 192 0 10856 1538191632 1 3 63 138 191 0 10865 1538191632 2 1 64 137 190 0 10874 1538191632 3 2 65 136 189 0 10883 1538191633 4 3 66 135 187 0 10892 1538191633 1 1 67 134 186 0 10901 1538191633 2 2 68 133 184 0 10910 1538191633 3 3 69 132 182 0 10919 1538191634 4 1 70 131 180 0 10928 1538191634 1 2 71 130 179 0 10937 1538191634 2 3 72 129 177 0 10946 1538191634 3 1 73 128 175 0 10955 1538191635 4 2 74 127 172 0 10964 1538191635 1 3 75 126 170 0 10973 1538191635 2 1 76 125 168 0 10982 1538191635 3 2 77 124 166 0 10991 1538191636 4 3 78 123 163 0 11000 1538191636 1 1 79 122 161 0 11009 1538191636 2 2 80 121 158 0 11018 1538191636 3 3 81 120 156 0 11027 1538191637 4 1 82 119 153 0 11036 1538191637 1 2 83 118 150 0 11045 1538191637 2 3 84 117 148 0 11054 1538191637 3 1 85 116 145 0 11063 1538191638 4 2 86 115 142 0 11072 1538191638 1 3 87 114 139 0 11081 1538191638 2 1 88 113 136 0 11090 1538191638 3 2 89 112 133 0 11099 1538191639 4 3 90 111 130 0 11108 1538191639 1 1 91 110 127 0 11117 1538191639 2 2 92 109 124 0 11126 1538191639 3 3 93 108 121 0 11135 1538191640 4 1 94 107 118 0 11144 1538191640 1 2 95 106 115 0 11153 1538191640 2 3 96 105 112 0 11162 1538191640 3 1 97 104 109 0 11171 1538191641 4 2 98 103 106 0 11180 1538191641 1 3 99 102 103 0 11189 1538191641 2 1 100 101 100 0 11198 1538191641 3 2 101 100 97 0 11207 1538191642 4 3 102 99 94 0 11216 1538191642 1 1 103 98 91 0 11225 1538191642 2 2 104 97 88 0 11234 1538191642 3 3 105 96 85 0 11243 1538191643 4 1 106 95 82 0 11252 1538191643 1 2 107 94 79 0 11261 1538191643 2 3 108 93 76 0 11270 1538191643 3 1 109 92 73 0 11279 1538191644 4 2 110 91 70 0 11288 1538191644 1 3 111 90 67 0 11297 1538191644 2 1 112 89 64 0 11306 1538191644 3 2 113 88 61 0 11315 1538191645 4 3 114 87 58 0 11324 1538191645 1 1 115 86 55 0 11333 1538191645 2 2 116 85 52 0 11342 1538191645 3 3 117 84 50 0 11351 1538191646 4 1 118 83 47 0 11360 1538191646 1 2 119 82 44 0 11369 1538191646 2 3 120 81 42 0 11378 1538191646 3 1 121 80 39 0 11387 1538191647 4 2 122 79 37 0 11396 1538191647 1 3 123 78 34 0 11405 1538191647 2 1 124 77 32 0 11414 1538191647 3 2 125 76 30 0 11423 1538191648 4 3 126 75 28 0 11432 1538191648 1 1 127 74 25 0 11441 1538191648 2 2 128 73 23 0 11450 1538191648 3 3 129 72 21 0 11459 1538191649 4 1 130 71 20 0 11468 1538191649 1 2 131 70 18 0 11477 1538191649 2 3 132 69 16 0 11486 1538191649 3 1 133 68 14 0 11495 1538191650 4 2 134 67 13 0 11504 1538191650 1 3 135 66 11 0 11513 1538191650 2 1 136 65 10 0 11522 1538191650 3 2 137 64 9 0 11531 1538191651 4 3 138 63 8 0 11540 1538191651 1 1 139 62 6 0 11549 1538191651 2 2 140 61 5 0 11558 1538191651 3 3 141 60 4 0 11567 1538191652 4 1 142 59 4 0 11576 1538191652 1 2 143 58 3 0 11585 1538191652 2 3 144 57 2 0 11594 1538191652 3 1 145 56 2 0 11603 1538191653 4 2 146 55 1 0 11612 1538191653 1 3 147 54 1 0 11621 1538191653 2 1 148 53 1 0 11630 1538191653 3 2 149 52 1 0 11639 1538191654 4 3 150 51 0 0 11648 1538191654 1 1 151 50 1 0 11657 1538191654 2 2 152 49 1 0 11666 1538191654 3 3 153 48 1 0 11675 1538191655 4 1 154 47 1 0 11684 1538191655 1 2 155 46 2 0 11693 1538191655 2 3 156 45 2 0 11702 1538191655 3 1 157 44 3 0 11711 1538191656 4 2 158 43 4 0 11720 1538191656 1 3 159 42 4 0 11729 1538191656 2 1 160 41 5 0 11738 1538191656 3 2 161 40 6 0 11747 1538191657 4 3 162 39 8 0 11756 1538191657 1 1 163 38 9 0 11765 1538191657 2 2 164 37 10 0 11774 1538191657 3 3 165 36 11 0 11783 1538191658 4 1 166 35 13 0 11792 1538191658 1 2 167 34 14 0 11801 1538191658 2 3 168 33 16 0 11810 1538191658 3 1 169 32 18 0 11819 1538191659 4 2 170 31 20 0 11828 1538191659 1 3 171 30 21 0 11837 1538191659 2 1 172 29 23 0 11846 1538191659 3 2 173 28 25 0 11855 1538191660 4 3 174 27 28 0 11864 1538191660 1 1 175 26 30 0 11873 1538191660 2 2 176 25 32 0 11882 1538191660 3 3 177 24 34 0 11891 1538191661 4 1 178 23 37 0 11900 1538191661 1 2 179 22 39 0 11909 1538191661 2 3 180 21 42 0 11918 1538191661 3 1 181 20 44 0 11927 1538191662 4 2 182 19 47 0 11936 1538191662 1 3 183 18 50 0 11945 1538191662 2 1 184 17 52 0 11954 1538191662 3 2 185 16 55 0 11963 1538191663 4 3 186 15 58 0 11972 1538191663 1 1 187 14 61 0 11981 1538191663 2 2 188 13 64 0 11990 1538191663 3 3 189 12 67 0 11999 1538191664 4 1 190 11 70 0 12008 1538191664 1 2 191 10 73 0 12017 1538191664 2 3 192 9 76 0 12026 1538191664 3 1 193 8 79 0 12035 1538191665 4 2 194 7 82 0 12044 1538191665 1 3 195 6 85 0 12053 1538191665 2 1 196 5 88 0 12062 1538191665 3 2 197 4 91 0 12071 1538191666 4 3 198 3 94 0 12080 1538191666 1 1 199 2 97 0 12089 1538191666 2 2 200 1 100 0 12098 1538191666 3 3 0 0 100 0 12107 1538191667 4 1 1 200 103 0 12116 1538191667 1 2 2 199 106 0 12125 1538191667 2 3 3 198 109 0 12134 1538191667 3 1 4 197 112 0 12143 1538191668 4 2 5 196 115 0 12152 1538191668 1 3 6 195 118 0 12161 1538191668 2 1 7 194 121 0 12170 1538191668 3 2 8 193 124 0 12179 1538191669 4 3 9 192 127 0 12188 1538191669 1 1 10 191 130 0 12197 1538191669 2 2 11 190 133 0 12206 1538191669 3 3 12 189 136 0 12215 1538191670 4 1 13 188 139 0 12224 1538191670 1 2 14 187 142 0 12233 1538191670 2 3 15 186 145 0 12242 1538191670 3 1 16 185 148 0 12251 1538191671 4 2 17 184 150 0 12260 1538191671 1 3 18 183 153 0 12269 1538191671 2 1 19 182 156 0 12278 1538191671 3 2 20 181 158 0 12287 1538191672 4 3 21 180 161 0 12296 1538191672 1 1 22 179 163 0 12305 1538191672 2 2 23 178 166 0 12314 1538191672 3 3 24 177 168 0 12323 1538191673 4 1 25 176 170 0 12332 1538191673 1 2 26 175 172 0 12341 1538191673 2 3 27 174 175 0 12350 1538191673 3 1 28 173 177 0 12359 1538191674 4 2 29 172 179 0 12368 1538191674 1 3 30 171 180 0 12377 1538191674 2 1 31 170 182 0 12386 1538191674 3 2 32 169 184 0 12395 1538191675 4 3 33 168 186 0 12404 1538191675 1 1 34 167 187 0 12413 1538191675 2 2 35 166 189 0 12422 1538191675 3 3 36 165 190 0 12431 1538191676 4 1 37 164 191 0 12440 1538191676 1 2 38 163 192 0 12449 1538191676 2 3 39 162 194 0 12458 1538191676 3 1 40 161 195 0 12467 1538191677 4 2 41 160 196 0 12476 1538191677 1 3 42 159 196 0 12485 1538191677 2 1 43 158 197 0 12494 1538191677 3 2 44 157 198 0 12503 1538191678 4 3 45 156 198 0 12512 1538191678 1 1 46 155 199 0 12521 1538191678 2 2 47 154 199 0 12530 1538191678 3 3 48 153 199 0 12539 1538191679 4 1 49 152 199 0 12548 1538191679 1 2 50 151 200 0 12557 1538191679 2 3 51 150 199 0 12566 1538191679 3 1 52 149 199 0 12575 1538191680 4 2 53 148 199 0 12584 1538191680 1 3 54 147 199 0 12593 1538191680 2 1 55 146 198 0 12602 1538191680 3 2 56 145 198 0 12611 1538191681 4 3 57 144 197 0 12620 1538191681 1 1 58 143 196 0 12629 1538191681 2 2 59 142 196 0 12638 1538191681 3 3 60 141 195 0 12647 1538191682 4 1 61 140 194 0 12656 1538191682 1 2 62 139 192 0 12665 1538191682 2 3 63 138 191 0 12674 1538191682 3 1 64 137 190 0 12683 1538191683 4 2 65 136 189 0 12692 1538191683 1 3 66 135 187 0 12701 1538191683 2 1 67 134 186 0 12710 1538191683 3 2 68 133 184 0 12719 1538191684 4 3 69 132 182 0 12728 1538191684 1 1 70 131 180 0 12737 1538191684 2 2 71 130 179 0 12746 1538191684 3 3 72 129 177 0 12755 1538191685 4 1 73 128 175 0 12764 1538191685 1 2 74 127 172 0 12773 1538191685 2 3 75 126 170 0 12782 1538191685 3 1 76 125 168 0 12791 1538191686 4 2 77 124 166 0 12800 1538191686 1 3 78 123 163 0 12809 1538191686 2 1 79 122 161 0 12818 1538191686 3 2 80 121 158 0 12827 1538191687 4 3 81 120 156 0 12836 1538191687 1 1 82 119 153 0 12845 1538191687 2 2 83 118 150 0 12854 1538191687 3 3 84 117 148 0 12863 1538191688 4 1 85 116 145 0 12872 1538191688 1 2 86 115 142 0 12881 1538191688 2 3 87 114 139 0 12890 1538191688 3 1 88 113 136 0 12899 1538191689 4 2 89 112 133 0 12908 1538191689 1 3 90 111 130 0 12917 1538191689 2 1 91 110 127 0 12926 1538191689 3 2 92 109 124 0 12935 1538191690 4 3 93 108 121 0 12944 1538191690 1 1 94 107 118 0 12953 1538191690 2 2 95 106 115 0 12962 1538191690 3 3 96 105 112 0 12971 1538191691 4 1 97 104 109 0 12980 1538191691 1 2 98 103 106 0 12989 1538191691 2 3 99 102 103 0 12998 1538191691 3 1 100 101 100 0 13007 1538191692 4 2 101 100 97 0 13016 1538191692 1 3 102 99 94 0 13025 1538191692 2 1 103 98 91 0 13034 1538191692 3 2 104 97 88 0 13043 1538191693 4 3 105 96 85 0 13052 1538191693 1 1 106 95 82 0 13061 1538191693 2 2 107 94 79 0 13070 1538191693 3 3 108 93 76 0 13079 1538191694 4 1 109 92 73 0 13088 1538191694 1 2 110 91 70 0 13097 1538191694 2 3 111 90 67 0 13106 1538191694 3 1 112 89 64 0 13115 1538191695 4 2 113 88 61 0 13124 1538191695 1 3 114 87 58 0 13133 1538191695 2 1 115 86 55 0 13142 1538191695 3 2 116 85 52 0 13151 1538191696 4 3 117 84 50 0 13160 1538191696 1 1 118 83 47 0 13169 1538191696 2 2 119 82 44 0 13178 1538191696 3 3 120 81 42 0 13187 1538191697 4 1 121 80 39 0 13196 1538191697 1 2 122 79 37 0 13205 1538191697 2 3 123 78 34 0 13214 1538191697 3 1 124 77 32 0 13223 1538191698 4 2 125 76 30 0 13232 1538191698 1 3 126 75 28 0 13241 1538191698 2 1 127 74 25 0 13250 1538191698 3 2 128 73 23 0 13259 1538191699 4 3 129 72 21 0 13268 1538191699 1 1 130 71 20 0 13277 1538191699 2 2 131 70 18 0 13286 1538191699 3 3 132 69 16 0 13295 1538191700 4 1 133 68 14 0 13304 1538191700 1 2 134 67 13 0 13313 1538191700 2 3 135 66 11 0 13322 1538191700 3 1 136 65 10 0 13331 1538191701 4 2 137 64 9 0 13340 1538191701 1 3 138 63 8 0 13349 1538191701 2 1 139 62 6 0 13358 1538191701 3 2 140 61 5 0 13367 1538191702 4 3 141 60 4 0 13376 1538191702 1 1 142 59 4 0 13385 1538191702 2 2 143 58 3 0 13394 1538191702 3 3 144 57 2 0 13403 1538191703 4 1 145 56 2 0 13412 1538191703 1 2 146 55 1 0 13421 1538191703 2 3 147 54 1 0 13430 1538191703 3 1 148 53 1 0 13439 1538191704 4 2 149 52 1 0 13448 1538191704 1 3 150 51 0 0 13457 1538191704 2 1 151 50 1 0 13466 1538191704 3 2 152 49 1 0 13475 1538191705 4 3 153 48 1 0 13484 1538191705 1 1 154 47 1 0 13493 1538191705 2 2 155 46 2 0 13502 1538191705 3 3 156 45 2 0 13511 1538191706 4 1 157 44 3 0 13520 1538191706 1 2 158 43 4 0 13529 1538191706 2 3 159 42 4 0 13538 1538191706 3 1 160 41 5 0 13547 1538191707 4 2 161 40 6 0 13556 1538191707 1 3 162 39 8 0 13565 1538191707 2 1 163 38 9 0 13574 1538191707 3 2 164 37 10 0 13583 1538191708 4 3 165 36 11 0 13592 1538191708 1 1 166 35 13 0 13601 1538191708 2 2 167 34 14 0 13610 1538191708 3 3 168 33 16 0 13619 1538191709 4 1 169 32 18 0 13628 1538191709 1 2 170 31 20 0 13637 1538191709 2 3 171 30 21 0 13646 1538191709 3 1 172 29 23 0 13655 1538191710 4 2 173 28 25 0 13664 1538191710 1 3 174 27 28 0 13673 1538191710 2 1 175 26 30 0 13682 1538191710 3 2 176 25 32 0 13691 1538191711 4 3 177 24 34 0 13700 1538191711 1 1 178 23 37 0 13709 1538191711 2 2 179 22 39 0 13718 1538191711 3 3 180 21 42 0 13727 1538191712 4 1 181 20 44 0 13736 1538191712 1 2 182 19 47 0 13745 1538191712 2 3 183 18 50 0 13754 1538191712 3 1 184 17 52 0 13763 1538191713 4 2 185 16 55 0 13772 1538191713 1 3 186 15 58 0 13781 1538191713 2 1 187 14 61 0 13790 1538191713 3 2 188 13 64 0 13799 1538191714 4 3 189 12 67 0 13808 1538191714 1 1 190 11 70 0 13817 1538191714 2 2 191 10 73 0 13826 1538191714 3 3 192 9 76 0 13835 1538191715 4 1 193 8 79 0 13844 1538191715 1 2 194 7 82 0 13853 1538191715 2 3 195 6 85 0 13862 1538191715 3 1 196 5 88 0 13871 1538191716 4 2 197 4 91 0 13880 1538191716 1 3 198 3 94 0 13889 1538191716 2 1 199 2 97 0 13898 1538191716 3 2 200 1 100 0 13907 1538191717 4 3 0 0 100 0 13916 1538191717 1 1 1 200 103 0 13925 1538191717 2 2 2 199 106 0 13934 1538191717 3 3 3 198 109 0 13943 1538191718 4 1 4 197 112 0 13952 1538191718 1 2 5 196 115 0 13961 1538191718 2 3 6 195 118 0 13970 1538191718 3 1 7 194 121 0 13979 1538191719 4 2 8 193 124 0 13988 1538191719 1 3 9 192 127 0 13997 1538191719 2 1 10 191 130 0 14006 1538191719 3 2 11 190 133 0 14015 1538191720 4 3 12 189 136 0 14024 1538191720 1 1 13 188 139 0 14033 1538191720 2 2 14 187 142 0 14042 1538191720 3 3 15 186 145 0 14051 1538191721 4 1 16 185 148 0 14060 1538191721 1 2 17 184 150 0 14069 1538191721 2 3 18 183 153 0 14078 1538191721 3 1 19 182 156 0 14087 1538191722 4 2 20 181 158 0 14096 1538191722 1 3 21 180 161 0 14105 1538191722 2 1 22 179 163 0 14114 1538191722 3 2 23 178 166 0 14123 1538191723 4 3 24 177 168 0 14132 1538191723 1 1 25 176 170 0 14141 1538191723 2 2 26 175 172 0 14150 1538191723 3 3 27 174 175 0 14159 1538191724 4 1 28 173 177 0 14168 1538191724 1 2 29 172 179 0 14177 1538191724 2 3 30 171 180 0 14186 1538191724 3 1 31 170 182 0 14195 1538191725 4 2 32 169 184 0 14204 1538191725 1 3 33 168 186 0 14213 1538191725 2 1 34 167 187 0 14222 1538191725 3 2 35 166 189 0 14231 1538191726 4 3 36 165 190 0 14240 1538191726 1 1 37 164 191 0 14249 1538191726 2 2 38 163 192 0 14258 1538191726 3 3 39 162 194 0 14267 1538191727 4 1 40 161 195 0 14276 1538191727 1 2 41 160 196 0 14285 1538191727 2 3 42 159 196 0 14294 1538191727 3 1 43 158 197 0 14303 1538191728 4 2 44 157 198 0 14312 1538191728 1 3 45 156 198 0 14321 1538191728 2 1 46 155 199 0 14330 1538191728 3 2 47 154 199 0 14339 1538191729 4 3 48 153 199 0 14348 1538191729 1 1 49 152 199 0 14357 1538191729 2 2 50 151 200 0 14366 1538191729 3 3 51 150 199 0 14375 1538191730 4 1 52 149 199 0 14384 1538191730 1 2 53 148 199 0 14393 1538191730 2 3 54 147 199 0 14402 1538191730 3 1 55 146 198 0 14411 1538191731 4 2 56 145 198 0 14420 1538191731 1 3 57 144 197 0 14429 1538191731 2 1 58 143 196 0 14438 1538191731 3 2 59 142 196 0 14447 1538191732 4 3 60 141 195 0 14456 1538191732 1 1 61 140 194 0 14465 1538191732 2 2 62 139 192 0 14474 1538191732 3 3 63 138 191 0 14483 1538191733 4 1 64 137 190 0 14492 1538191733 1 2 65 136 189 0 14501 1538191733 2 3 66 135 187 0 14510 1538191733 3 1 67 134 186 0 14519 1538191734 4 2 68 133 184 0 14528 1538191734 1 3 69 132 182 0 14537 1538191734 2 1 70 131 180 0 14546 1538191734 3 2 71 130 179 0 14555 1538191735 4 3 72 129 177 0 14564 1538191735 1 1 73 128 175 0 14573 1538191735 2 2 74 127 172 0 14582 1538191735 3 3 75 126 170 0 14591 1538191736 4 1 76 125 168 0 14600 1538191736 1 2 77 124 166 0 14609 1538191736 2 3 78 123 163 0 14618 1538191736 3 1 79 122 161 0 14627 1538191737 4 2 80 121 158 0 Port open Initializing RTC... Initializing FRAM... Found I2C FRAM read nextFramWriteAddr 14636 from address 0 Using interrupt number 5, pin 18 Clearing 2000 FRAM addresses... Setting NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION (0) to FIRST_PKT_STORAGE_ADDR (2) Displaying memory locations 0 to 2000 0x0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x10: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x20: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x30: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x40: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x50: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x60: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x70: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x80: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x90: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0xA0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0xB0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0xC0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0xD0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0xE0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0xF0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x100: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x110: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x120: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x130: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x140: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x150: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x160: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x170: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x180: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x190: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x1A0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x1B0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x1C0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x1D0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x1E0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x1F0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x200: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x210: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x220: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x230: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x240: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x250: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x260: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x270: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x280: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x290: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x2A0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x2B0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x2C0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x2D0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x2E0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x2F0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x300: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x310: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x320: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x330: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x340: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x350: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x360: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x370: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x380: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x390: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x3A0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x3B0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x3C0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x3D0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x3E0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x3F0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x400: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x410: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x420: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x430: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x440: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x450: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x460: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x470: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x480: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x490: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x4A0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x4B0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x4C0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x4D0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x4E0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x4F0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x500: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x510: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x520: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x530: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x540: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x550: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x560: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x570: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x580: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x590: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x5A0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x5B0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x5C0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x5D0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x5E0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x5F0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x600: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x610: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x620: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x630: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x640: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x650: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x660: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x670: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x680: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x690: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x6A0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x6B0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x6C0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x6D0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x6E0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x6F0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x700: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x710: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x720: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x730: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x740: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x750: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x760: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x770: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x780: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x790: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x7A0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x7B0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x7C0: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 Enabling ISR, Entering Loop()addr unixtime mode submode Ldist Rdist Fdist batV 2 1538191746 1 1 1 200 103 0 11 1538191746 2 2 2 199 106 0 20 1538191747 3 3 3 198 109 0 29 1538191747 4 1 4 197 112 0 38 1538191747 1 2 5 196 115 0 displaying 5 packets, from address 2 to address 46 addr unixtime mode submode Ldist Rdist Fdist batV 2 1538191746 1 1 1 200 103 0 11 1538191746 2 2 2 199 106 0 20 1538191747 3 3 3 198 109 0 29 1538191747 4 1 4 197 112 0 38 1538191747 1 2 5 196 115 0 ---------------- Done!----------------- addr unixtime mode submode Ldist Rdist Fdist batV 47 1538191747 2 3 6 195 118 0 56 1538191748 3 1 7 194 121 0 65 1538191748 4 2 8 193 124 0 74 1538191748 1 3 9 192 127 0 83 1538191748 2 1 10 191 130 0 92 1538191749 3 2 11 190 133 0 101 1538191749 4 3 12 189 136 0 110 1538191749 1 1 13 188 139 0 119 1538191749 2 2 14 187 142 0 128 1538191750 3 3 15 186 145 0 137 1538191750 4 1 16 185 148 0 146 1538191750 1 2 17 184 150 0 155 1538191750 2 3 18 183 153 0 164 1538191751 3 1 19 182 156 0 173 1538191751 4 2 20 181 158 0 182 1538191751 1 3 21 180 161 0 191 1538191751 2 1 22 179 163 0 200 1538191752 3 2 23 178 166 0 209 1538191752 4 3 24 177 168 0 218 1538191752 1 1 25 176 170 0 227 1538191752 2 2 26 175 172 0 236 1538191753 3 3 27 174 175 0 245 1538191753 4 1 28 173 177 0 254 1538191753 1 2 29 172 179 0 263 1538191753 2 3 30 171 180 0 272 1538191754 3 1 31 170 182 0 displaying 26 packets, from address 47 to address 280 addr unixtime mode submode Ldist Rdist Fdist batV 47 1538191747 2 3 6 195 118 0 56 1538191748 3 1 7 194 121 0 65 1538191748 4 2 8 193 124 0 74 1538191748 1 3 9 192 127 0 83 1538191748 2 1 10 191 130 0 92 1538191749 3 2 11 190 133 0 101 1538191749 4 3 12 189 136 0 110 1538191749 1 1 13 188 139 0 119 1538191749 2 2 14 187 142 0 128 1538191750 3 3 15 186 145 0 137 1538191750 4 1 16 185 148 0 146 1538191750 1 2 17 184 150 0 155 1538191750 2 3 18 183 153 0 164 1538191751 3 1 19 182 156 0 173 1538191751 4 2 20 181 158 0 182 1538191751 1 3 21 180 161 0 191 1538191751 2 1 22 179 163 0 200 1538191752 3 2 23 178 166 0 209 1538191752 4 3 24 177 168 0 218 1538191752 1 1 25 176 170 0 227 1538191752 2 2 26 175 172 0 236 1538191753 3 3 27 174 175 0 245 1538191753 4 1 28 173 177 0 254 1538191753 1 2 29 172 179 0 263 1538191753 2 3 30 171 180 0 272 1538191754 3 1 31 170 182 0 ---------------- Done!----------------- addr unixtime mode submode Ldist Rdist Fdist batV 281 1538191754 4 2 32 169 184 0 290 1538191754 1 3 33 168 186 0 displaying 2 packets, from address 281 to address 298 addr unixtime mode submode Ldist Rdist Fdist batV 281 1538191754 4 2 32 169 184 0 290 1538191754 1 3 33 168 186 0 ---------------- Done!----------------- addr unixtime mode submode Ldist Rdist Fdist batV 299 1538191754 2 1 34 167 187 0 308 1538191755 3 2 35 166 189 0 317 1538191755 4 3 36 165 190 0 326 1538191755 1 1 37 164 191 0 335 1538191755 2 2 38 163 192 0 344 1538191756 3 3 39 162 194 0 353 1538191756 4 1 40 161 195 0 362 1538191756 1 2 41 160 196 0 371 1538191756 2 3 42 159 196 0 380 1538191757 3 1 43 158 197 0 389 1538191757 4 2 44 157 198 0 398 1538191757 1 3 45 156 198 0 407 1538191757 2 1 46 155 199 0 416 1538191758 3 2 47 154 199 0 425 1538191758 4 3 48 153 199 0 434 1538191758 1 1 49 152 199 0 443 1538191758 2 2 50 151 200 0 452 1538191759 3 3 51 150 199 0 461 1538191759 4 1 52 149 199 0 470 1538191759 1 2 53 148 199 0 479 1538191759 2 3 54 147 199 0 488 1538191760 3 1 55 146 198 0 497 1538191760 4 2 56 145 198 0 506 1538191760 1 3 57 144 197 0 515 1538191760 2 1 58 143 196 0 524 1538191761 3 2 59 142 196 0 533 1538191761 4 3 60 141 195 0 542 1538191761 1 1 61 140 194 0 551 1538191761 2 2 62 139 192 0 560 1538191762 3 3 63 138 191 0 569 1538191762 4 1 64 137 190 0 578 1538191762 1 2 65 136 189 0 587 1538191762 2 3 66 135 187 0 596 1538191763 3 1 67 134 186 0 605 1538191763 4 2 68 133 184 0 614 1538191763 1 3 69 132 182 0 623 1538191763 2 1 70 131 180 0 632 1538191764 3 2 71 130 179 0 641 1538191764 4 3 72 129 177 0 650 1538191764 1 1 73 128 175 0 659 1538191764 2 2 74 127 172 0 668 1538191765 3 3 75 126 170 0 677 1538191765 4 1 76 125 168 0 686 1538191765 1 2 77 124 166 0 695 1538191765 2 3 78 123 163 0 704 1538191766 3 1 79 122 161 0 713 1538191766 4 2 80 121 158 0 722 1538191766 1 3 81 120 156 0 731 1538191766 2 1 82 119 153 0 740 1538191767 3 2 83 118 150 0 749 1538191767 4 3 84 117 148 0 758 1538191767 1 1 85 116 145 0 767 1538191767 2 2 86 115 142 0 776 1538191768 3 3 87 114 139 0 785 1538191768 4 1 88 113 136 0 794 1538191768 1 2 89 112 133 0 803 1538191768 2 3 90 111 130 0 812 1538191769 3 1 91 110 127 0 821 1538191769 4 2 92 109 124 0 830 1538191769 1 3 93 108 121 0 839 1538191769 2 1 94 107 118 0 848 1538191770 3 2 95 106 115 0 857 1538191770 4 3 96 105 112 0 866 1538191770 1 1 97 104 109 0 displaying 64 packets, from address 299 to address 874 addr unixtime mode submode Ldist Rdist Fdist batV 299 1538191754 2 1 34 167 187 0 308 1538191755 3 2 35 166 189 0 317 1538191755 4 3 36 165 190 0 326 1538191755 1 1 37 164 191 0 335 1538191755 2 2 38 163 192 0 344 1538191756 3 3 39 162 194 0 353 1538191756 4 1 40 161 195 0 362 1538191756 1 2 41 160 196 0 371 1538191756 2 3 42 159 196 0 380 1538191757 3 1 43 158 197 0 389 1538191757 4 2 44 157 198 0 398 1538191757 1 3 45 156 198 0 407 1538191757 2 1 46 155 199 0 416 1538191758 3 2 47 154 199 0 425 1538191758 4 3 48 153 199 0 434 1538191758 1 1 49 152 199 0 443 1538191758 2 2 50 151 200 0 452 1538191759 3 3 51 150 199 0 461 1538191759 4 1 52 149 199 0 470 1538191759 1 2 53 148 199 0 479 1538191759 2 3 54 147 199 0 488 1538191760 3 1 55 146 198 0 497 1538191760 4 2 56 145 198 0 506 1538191760 1 3 57 144 197 0 515 1538191760 2 1 58 143 196 0 524 1538191761 3 2 59 142 196 0 533 1538191761 4 3 60 141 195 0 542 1538191761 1 1 61 140 194 0 551 1538191761 2 2 62 139 192 0 560 1538191762 3 3 63 138 191 0 569 1538191762 4 1 64 137 190 0 578 1538191762 1 2 65 136 189 0 587 1538191762 2 3 66 135 187 0 596 1538191763 3 1 67 134 186 0 605 1538191763 4 2 68 133 184 0 614 1538191763 1 3 69 132 182 0 623 1538191763 2 1 70 131 180 0 632 1538191764 3 2 71 130 179 0 641 1538191764 4 3 72 129 177 0 650 1538191764 1 1 73 128 175 0 659 1538191764 2 2 74 127 172 0 668 1538191765 3 3 75 126 170 0 677 1538191765 4 1 76 125 168 0 686 1538191765 1 2 77 124 166 0 695 1538191765 2 3 78 123 163 0 704 1538191766 3 1 79 122 161 0 713 1538191766 4 2 80 121 158 0 722 1538191766 1 3 81 120 156 0 731 1538191766 2 1 82 119 153 0 740 1538191767 3 2 83 118 150 0 749 1538191767 4 3 84 117 148 0 758 1538191767 1 1 85 116 145 0 767 1538191767 2 2 86 115 142 0 776 1538191768 3 3 87 114 139 0 785 1538191768 4 1 88 113 136 0 794 1538191768 1 2 89 112 133 0 803 1538191768 2 3 90 111 130 0 812 1538191769 3 1 91 110 127 0 821 1538191769 4 2 92 109 124 0 830 1538191769 1 3 93 108 121 0 839 1538191769 2 1 94 107 118 0 848 1538191770 3 2 95 106 115 0 857 1538191770 4 3 96 105 112 0 866 1538191770 1 1 97 104 109 0 ---------------- Done!----------------- addr unixtime mode submode Ldist Rdist Fdist batV 875 1538191771 2 2 98 103 106 0 884 1538191771 3 3 99 102 103 0 893 1538191771 4 1 100 101 100 0 902 1538191771 1 2 101 100 97 0 911 1538191771 2 3 102 99 94 0 920 1538191772 3 1 103 98 91 0 929 1538191772 4 2 104 97 88 0 938 1538191772 1 3 105 96 85 0 947 1538191772 2 1 106 95 82 0 956 1538191773 3 2 107 94 79 0 965 1538191773 4 3 108 93 76 0 974 1538191773 1 1 109 92 73 0 983 1538191773 2 2 110 91 70 0 992 1538191774 3 3 111 90 67 0 1001 1538191774 4 1 112 89 64 0 1010 1538191774 1 2 113 88 61 0 1019 1538191774 2 3 114 87 58 0 1028 1538191775 3 1 115 86 55 0 1037 1538191775 4 2 116 85 52 0 1046 1538191775 1 3 117 84 50 0 1055 1538191775 2 1 118 83 47 0 1064 1538191776 3 2 119 82 44 0 1073 1538191776 4 3 120 81 42 0 1082 1538191776 1 1 121 80 39 0 1091 1538191776 2 2 122 79 37 0 1100 1538191777 3 3 123 78 34 0 Port closed |
And here is an Excel plot showing the simulated values
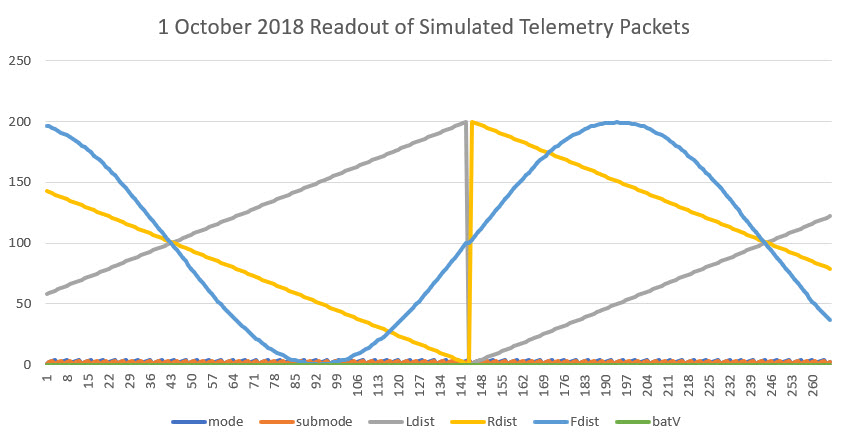
Plot of the simulated values generated by the test program
So now I have a way for Wall-E2 to write a minute-by-minute diary of its operating state to non-volatile storage, but I don’t yet have a good way to read it all back out again. That’s the next step – stay tuned!
01 October Update:
I created a small program to read back telemetry packets from FRAM. When I want to see what Wall-E2 has been up to, I will replace his normal operating firmware with this sketch, which will allow me to read out all or parts of FRAM contents. The sketch is included below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 |
/* Name: FramPacketReadout.ino Created: 9/29/2018 7:01:55 PM Author: DESKTOP-0KFED77\Frank The purpose of this project is to develop the code needed to display telemetry packets from Wall-E2, stored in encoded form in the onboard FRAM. The idea is to read out the packets with the timestamp in human-readable form, along with the other information. The stored telemetry packet is formatted as a 10-byte packet: Time: 4-bytes mode/submode: 1 byte left distance: 1 byte right distance: 1 byte forward distance: 1 byte battery voltage above 5V: 1 byte This project makes use of the CFRAMStatePacket class, which is completely defined in FramPacket.h (no .cpp file - just the .h). This class contains methods to read and write telemetry packets from/to an Adafruit FRAM breakout board, accessible through the Adafruit_FRAM_I2C library To use the CFRAMStatePacket class, an object is instantiated using a constructor that takes a single parameter - the address of the Adafruit_FRAM_I2C FRAM driver object. This pointer is then used for all interactions with the FRAM. */ #include <Wire.h> #include "Adafruit_FRAM_I2C.h" #include <time.h>; #include <PrintEx.h> #include <elapsedMillis/elapsedMillis.h> #include <Time-master/time.h> #include <avr/sleep.h> //needed for sleep() functions //#include <math.h> StreamEx mySerial = Serial; elapsedMillis mSecSinceLastPacketWrite; Adafruit_FRAM_I2C fram = Adafruit_FRAM_I2C(); const int FIRST_PKT_STORAGE_ADDR = 2;//addr 0/1 reserved for nextFramWriteAddr //const int FIRST_PKT_STORAGE_ADDR = 32000;//09/29/18 for rollover debug const int NEXTFRAMWRITEADDR_FRAMSTORAGELOCATION = 0; //the next free address is written to this address const int LAST_AVAIL_PACKET_WRITE_ADDR = 32759; //last packet write address avail in 256kb FRAM int ReadStartAddress = FIRST_PKT_STORAGE_ADDR; //writing starts with this address int ReadStopAddress = LAST_AVAIL_PACKET_WRITE_ADDR; //FramPacket.h holds 'inline' definition of CFRAMStatePacket class //this has to come after #include "Adafruit_FRAM_I2C.h" and Adafruit_FRAM_I2C fram = Adafruit_FRAM_I2C(); #include "FramPacket.h" CFRAMStatePacket packet = CFRAMStatePacket(&fram); //this object is used for all FRAM transactions //fwd declaration required to avoid '... does not name a type' error CFRAMStatePacket GetNextSimFRAMPacket(CFRAMStatePacket pkt); //char daysOfTheWeek[7][12] = { "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" }; char Instr[20]; int packetsize = CFRAMStatePacket::packet_size; void setup() { Serial.begin(115200); //initialize FRAM - this reads/checks FRAM device IDs to confirm valid connection mySerial.printf("Initializing FRAM...\n"); if (fram.begin()) // you can stick the new i2c addr in here, e.g. begin(0x51); { Serial.println("Found I2C FRAM"); } else { Serial.println("I2C FRAM not identified ... check your connections?\r\n"); Serial.println("Will continue in case this processor doesn't support repeated start\r\n"); } //Display 4 undecoded packets from 2 through 29 to establish baseline DisplayFRAMPackets(2, 30); //do a test by reading just the first packet, and showing it to the user packet.Read(ReadStartAddress); packet.PrintHumanReadable(); //get start address for readout mySerial.printf("In Setup()\n"); ReadStartAddress = GetIntegerParameter("FRAM Readout Start Address (>= 2)", 2); ReadStopAddress = GetIntegerParameter("FRAM Readout Stop Address (<= 32759", LAST_AVAIL_PACKET_WRITE_ADDR); mySerial.printf("start/stop addresses are %d, %d\n", ReadStartAddress, ReadStopAddress); mySerial.printf("Entering print loop - send any key to terminate\n"); mySerial.printf("addr\tdate/time\t\t\tmode\tsubmode\tLdist\tRdist\tFdist\tbatV\n"); } void loop() { int readaddr = ReadStartAddress; Serial.flush(); char readbuf[80]; int numchars = Serial.available(); //mySerial.printf("There are %d chars available in serial channel\n", numchars); while (numchars > 0) { Serial.readBytes(readbuf, 80); //mySerial.printf("There are still %d chars available in serial channel\n", numchars); } while (readaddr <= ReadStopAddress && Serial.available() == 0) { //read, decode, and print a FRAM packet //mySerial.printf("Printing address %d....\n", readaddr); packet.Read(readaddr); mySerial.printf("%d\t", readaddr); //put address in 1st column packet.PrintHumanReadable();//prints date/time in human-readable form readaddr += packetsize; //check for user input if (Serial.available() > 0) { // read the incoming byte: int incomingByte = Serial.read(); // say what you got: Serial.print("I received: "); Serial.println(incomingByte, DEC); Serial.print("Quitting the program!"); delay(1000); cli(); sleep_enable(); sleep_cpu(); } } mySerial.printf("Finished printing requested FRAM addresses - have a nice day! ;-)\n"); delay(1000); cli(); sleep_enable(); sleep_cpu(); } int GetIntegerParameter(String prompt, int defaultval) { int param = 0; bool bDone = false; while (!bDone) { Serial.print(prompt); Serial.print(" ("); Serial.print(defaultval); Serial.print("): "); while (Serial.available() == 0); //waits for input String res = Serial.readString(); res.trim(); int reslen = res.length(); if (reslen == 0) //user entered CR only { bDone = true; param = defaultval; } else { res.toCharArray(Instr, reslen + 1); if (isNumeric(Instr)) { param = atoi(Instr); bDone = true; } else { Serial.print(Instr); Serial.println(" Is invalid input - please try again"); } } } Serial.println(param); return param; } // check a string to see if it is numeric and accept Decimal point //copied from defragster's post at https://forum.pjrc.com/threads/27842-testing-if-a-string-is-numeric bool isNumeric(char * str) { byte ii = 0; bool RetVal = false; if ('-' == str[ii]) ii++; while (str[ii]) { if ('.' == str[ii]) { ii++; break; } if (!isdigit(str[ii])) return false; ii++; RetVal = true; } while (str[ii]) { if (!isdigit(str[ii])) return false; ii++; RetVal = true; } return RetVal; } void DisplayFRAMPackets(int startaddr, int stopaddr) { //Purpose: Read complete state packets from the FRAM between the specified addresses // The telemetry packet is formatted as a 10 - byte packet : // Time: 4 - bytes // mode / submode : 1 byte // left distance : 1 byte // right distance : 1 byte // forward distance : 1 byte // battery voltage above 5V : 1 byte //Inputs: // nextreadaddr = 1st address to be read // nextwriteaddr = next available address for writes //Notes: // 09/28/18 c/o prevDispFramEndAddr = nextwriteaddr - 1; now done in calling pgm int numpackets = 1 + (stopaddr - startaddr - 1) / CFRAMStatePacket::packet_size; mySerial.printf("\ndisplaying %d packets, from address %d to address %d\n", numpackets, startaddr, stopaddr - 1); mySerial.printf("addr\tunixtime\t\tmode\tsubmode\tLdist\tRdist\tFdist\tbatV\n"); for (int i = 0; i < numpackets; i++) { mySerial.printf("%d\t", startaddr); //puts fram addr in front of each packet readout startaddr = packet.Read(startaddr); packet.Print(); } mySerial.printf("---------------- Done!-----------------\n\n"); } |
and a typical output run is shown below. Note that this program decodes the stored 4-byte unix time value into human-readable date/time format. And yes, I know it’s in that funny ‘American’ mm/dd/yyyy format, but I’m an American, so … ;-).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
Opening port Port open Initializing FRAM... Found I2C FRAM displaying 4 packets, from address 2 to address 29 addr unixtime mode submode Ldist Rdist Fdist batV 2 1538219039 1 1 58 143 196 0 11 1538219039 2 2 59 142 196 0 20 1538219039 3 3 60 141 195 0 29 1538219040 4 1 61 140 194 0 ---------------- Done!----------------- 11:03:59 09/29/2018 1 1 58 143 196 0 In Setup() FRAM Readout Start Address (>= 2) (2): 2 FRAM Readout Stop Address (<= 32759 (32759): 100 start/stop addresses are 2, 100 Entering print loop - send any key to terminate addr date/time mode submode Ldist Rdist Fdist batV 2 11:03:59 09/29/2018 1 1 58 143 196 0 11 11:03:59 09/29/2018 2 2 59 142 196 0 20 11:03:59 09/29/2018 3 3 60 141 195 0 29 11:04:00 09/29/2018 4 1 61 140 194 0 38 11:04:00 09/29/2018 1 2 62 139 192 0 47 11:04:00 09/29/2018 2 3 63 138 191 0 56 11:04:00 09/29/2018 3 1 64 137 190 0 65 11:04:01 09/29/2018 4 2 65 136 189 0 74 11:04:01 09/29/2018 1 3 66 135 187 0 83 11:04:01 09/29/2018 2 1 67 134 186 0 92 11:04:01 09/29/2018 3 2 68 133 184 0 Finished printing requested FRAM addresses - have a nice day! ;-) |
Frank